目次
参考リンク
YouTube

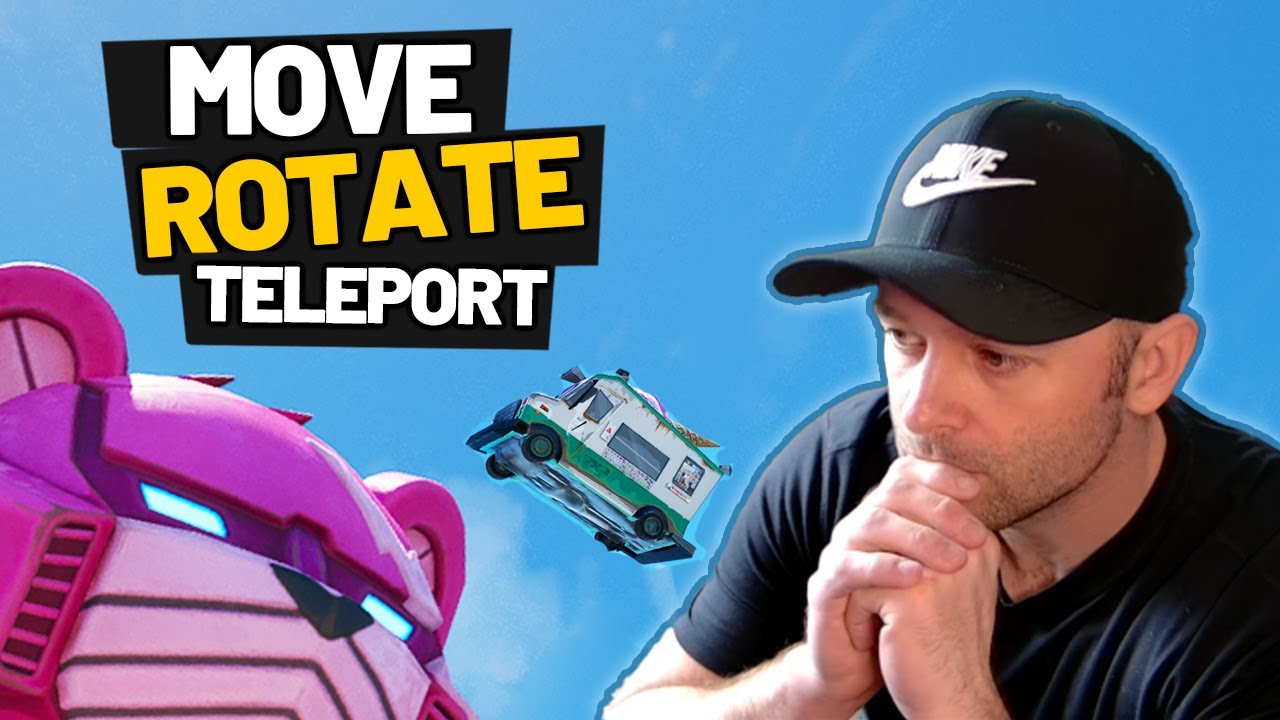
How To Move And Spin Props In UEFN With Verse
Want spinning coins and weapons and moving platforms? Watch this beginner tutorial for UEFN & VerseWHAT YOU’LL LEARN- Teleport (Teleporting props)- MoveTo (M…
- 他の動画もとても参考になる。
動かすもの
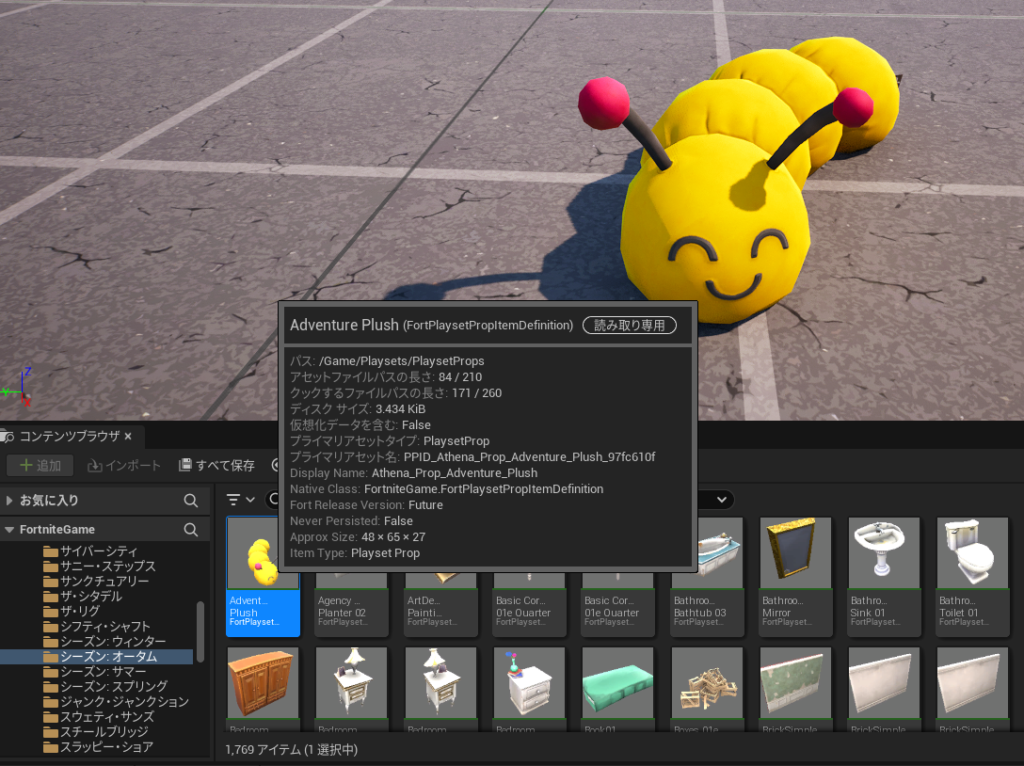
- 動画と同じ「AdventurePlush」を使用する。
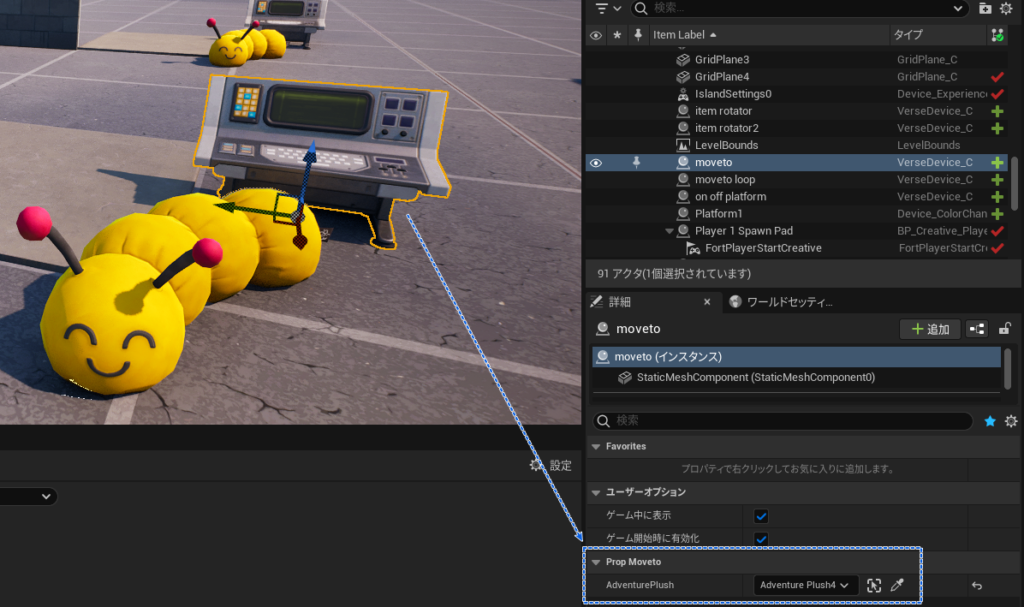
- Verseコードを新規作成したら「Verseコードをビルド」してレベル上にVerseのDeviceを配置、そして動かす「AdventurePlush」を設定するのを忘れないように。
TeleportToで動かす
using { /Fortnite.com/Devices }
using { /Verse.org/Simulation }
using { /UnrealEngine.com/Temporary/Diagnostics }
using { /UnrealEngine.com/Temporary/SpatialMath }
prop_teleport := class(creative_device):
@editable
var AdventurePlush : creative_prop = creative_prop{}
OnBegin<override>()<suspends>:void=
Sleep(5.0)
TereportProp(AdventurePlush)
TereportProp(Prop : creative_prop): void=
Transform := Prop.GetTransform()
PositionX := Transform.Translation.X
PositionY := Transform.Translation.Y
PositionZ := Transform.Translation.Z
Rotation := Transform.Rotation
NewRotation := Rotation.ApplyYaw(90.0)
NewPosition := vector3{X := PositionX, Y :=PositionY + 512.0, Z :=PositionZ + 384.0}
if (Prop.TeleportTo[NewPosition, NewRotation]):
Print("Teleport NewPosition: {NewPosition}")
Print("Teleport NewRotation: {NewRotation}")
- ゲームを開始すると5秒後に「AdventurePlush」がY軸方向にの床の幅1マス分(512)、Z軸方向に壁の高さ1マス分(384)移動する。
- 一瞬で移動。
コードを見る
using { /UnrealEngine.com/Temporary/SpatialMath }
- rotationの関数を使用するのに必要となるので冒頭に追記。
@editable
var AdventurePlush : creative_prop = creative_prop{}
- 動かすcreative_propを登録する。エディタで配置した「AdventurePlush」を設定する。
Sleep(5.0)
TereportProp(AdventurePlush)
- ゲーム開始して5秒後にTereportProp関数を読んで動かしている。
Transform := Prop.GetTransform()
PositionX := Transform.Translation.X
PositionY := Transform.Translation.Y
PositionZ := Transform.Translation.Z
Rotation := Transform.Rotation
- 配置した「AdventurePlush」の初期の位置と回転情報を取得する。
- X軸・Y軸・Z軸。回転それぞれ取得して変数に入れる。
NewRotation := Rotation.ApplyYaw(90.0)
NewPosition := vector3{X := PositionX, Y :=PositionY + 512.0, Z :=PositionZ + 384.0}
- 回転させる情報を設定する。初期の回転にApplyYawでYaw方向に90度加えた情報を変数に入れる。
- 移動させる情報を設定する。初期位置からX軸はそのまま、Y軸に512を加え、Z軸に384加えた情報を変数に入れる。
あわせて読みたい

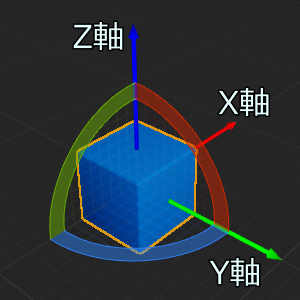
回転について[Rotation]
ロール、ピッチ、ヨー ロール(Roll)、ピッチ(Pitch)、ヨー(Yaw)とは、物体の3次元における姿勢を表すのに使用される回転の種類であり、航空機や船舶などの操縦に用いら…
if (Prop.TeleportTo[NewPosition, NewRotation]):
- 移動させる位置と回転情報を設定してTeleportTo関数を呼ぶ。
- TeleportToは失敗する可能性がある関数なので引数は [ ] で囲い、失敗コンテキストで呼ぶ必要があるのでif式を使用する。
Print("Teleport NewPosition: {NewPosition}")
Print("Teleport NewRotation: {NewRotation}")
- 移動後の位置と回転情報をログに表示。
MoveToで動かす
using { /Fortnite.com/Devices }
using { /Verse.org/Simulation }
using { /UnrealEngine.com/Temporary/Diagnostics }
using { /UnrealEngine.com/Temporary/SpatialMath }
prop_moveto := class(creative_device):
@editable
var AdventurePlush : creative_prop = creative_prop{}
OnBegin<override>()<suspends>:void=
Sleep(5.0)
spawn:
MoveProp(AdventurePlush)
MoveProp(Prop : creative_prop)<suspends>: void=
Transform := Prop.GetTransform()
PositionX := Transform.Translation.X
PositionY := Transform.Translation.Y
PositionZ := Transform.Translation.Z
Rotation := Transform.Rotation
NewRotation := Rotation.ApplyYaw(90.0)
NewPosition := vector3{X := PositionX, Y :=PositionY + 512.0, Z :=PositionZ + 384.0}
Prop.MoveTo(NewPosition, NewRotation, 2.0)
Print("Move NewPosition: {NewPosition}")
Print("Move NewRotation: {NewRotation}")
- ゲームを開始すると5秒後にcreative_propがY軸方向にの床の幅1マス分(512)、Z軸方向に壁の高さ1マス分(384)、2秒かけて移動する。
- 2秒かけてなめらかに移動。
コードを見る
OnBegin<override>()<suspends>:void=
Sleep(5.0)
spawn:
MoveProp(AdventurePlush)
MoveProp(Prop : creative_prop)<suspends>: void=
- MoveToは非同期関数なので、作成したMoveProp関数に<suspends>をつけている。
- 非同期関数MovePropを呼ぶのでspawn式を使用する。
Prop.MoveTo(NewPosition, NewRotation, 2.0)
- 移動させる位置と回転情報、移動の時間(秒)を設定してMoveTo関数を呼ぶ。
TeleportToで連続的に動かす
using { /Fortnite.com/Devices }
using { /Verse.org/Simulation }
using { /UnrealEngine.com/Temporary/Diagnostics }
using { /UnrealEngine.com/Temporary/SpatialMath }
prop_teleport_loop := class(creative_device):
@editable
var AdventurePlush : creative_prop = creative_prop{}
OnBegin<override>()<suspends>:void=
Sleep(5.0)
spawn:
TereportPropLoop(AdventurePlush)
TereportPropLoop(Prop : creative_prop)<suspends>: void=
loop:
Transform := Prop.GetTransform()
PositionX := Transform.Translation.X
PositionY := Transform.Translation.Y
PositionZ := Transform.Translation.Z
Rotation := Transform.Rotation
NewRotation := Rotation.ApplyYaw(0.01)
NewPosition := vector3{X := PositionX, Y :=PositionY + 1.0, Z :=PositionZ + 1.0}
if (Prop.TeleportTo[NewPosition, NewRotation]):
Sleep(0.01)
- loop処理でまわす。
- loop処理の時間をSleep()関数で設定する。非同期関数なので<suspends>をつけてspawnで呼んでいる。
- 動きがぎこちない。
MoveToで連続的に動かす
using { /Fortnite.com/Devices }
using { /Verse.org/Simulation }
using { /UnrealEngine.com/Temporary/Diagnostics }
using { /UnrealEngine.com/Temporary/SpatialMath }
prop_moveto_loop := class(creative_device):
@editable
var AdventurePlush : creative_prop = creative_prop{}
OnBegin<override>()<suspends>:void=
Sleep(5.0)
spawn:
MovePropLoop(AdventurePlush)
MovePropLoop(Prop : creative_prop)<suspends>: void=
Transform := Prop.GetTransform()
PositionX := Transform.Translation.X
PositionY := Transform.Translation.Y
PositionZ := Transform.Translation.Z
Rotation := Transform.Rotation
NewRotation := Rotation.ApplyYaw(90.0)
NewPosition := vector3{X := PositionX, Y :=PositionY + 100.0, Z :=PositionZ + 100.0}
MoveResult := Prop.MoveTo(NewPosition, NewRotation, 2.0)
if (MoveResult = move_to_result.DestinationReached):
MovePropLoop(Prop)
- MoveToの処理が終わったら自分MovePropLoop()を呼ぶ。
- 参考動画で記述していたMoveResult = move_to_result.DestinationReachedで目的地に着いたがどうかをチェックしているっぽい。
- なめらかに移動し続けている。
コメント